Best Practices for Writing Dockerfiles


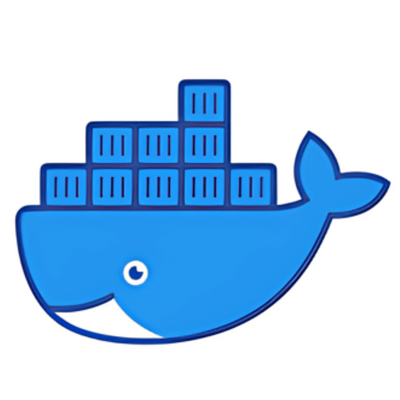
Docker has become an essential tool for developers and system administrators, providing a lightweight and portable way to package and distribute applications. Writing a Dockerfile is the first step in creating a Docker image, and following best practices can help ensure that your images are efficient, secure, and easy to maintain. In this article, we will explore some of the most important best practices for writing Dockerfiles, with in-depth technical explanations.
1. Use a minimal base image
The base image is the starting point for your Docker image, and it's important to choose one that is as small and minimal as possible. This will help reduce the size of your final image, which can improve build times and reduce storage costs.
For example, instead of using the ubuntu
base image, which includes a full Linux distribution, you can use the alpine
image, which is based on the lightweight Alpine Linux distribution. The alpine
image is typically much smaller than the ubuntu
image, which can result in faster build times and smaller image sizes.
FROM alpine:latest
2. Use multi-stage builds
Multi-stage builds allow you to use multiple base images in a single Dockerfile, which can help reduce the size of your final image and improve build times. With multi-stage builds, you can use one base image to compile your application, and then copy the compiled binary to a smaller base image for deployment.
For example, you can use the node:14-alpine
image to compile your Node.js application, and then copy the compiled binary to the alpine
image for deployment. Read more here about multi stage build.
# Build stage
FROM node:14-alpine as build
WORKDIR /app
COPY package.json yarn.lock ./
RUN yarn install
COPY . .
RUN yarn build
# Deploy stage
FROM alpine:latest
WORKDIR /app
COPY --from=build /app/dist ./dist
CMD ["node", "dist/index.js"]
3. Use the .dockerignore
file
The .dockerignore
file allows you to exclude files and directories from the build context, which can help reduce build times and improve security. By default, Docker includes the entire contents of the build context in the final image, which can result in large image sizes and potential security vulnerabilities.
For example, you can use the .dockerignore
file to exclude the node_modules
directory, which is not needed in the final image.
node_modules/
4. Use environment variables
Environment variables allow you to configure your application at runtime, without hardcoding values in your code. This can help improve security and make it easier to manage your application in different environments.
For example, you can use environment variables to configure the database connection string for your application.
ENV DATABASE_URL=postgresql://user:password@host:port/database
5. Use the COPY
command carefully
The COPY
command is used to copy files and directories from the build context to the Docker image. However, it's important to use it carefully, as it can result in large image sizes and potential security vulnerabilities.
For example, instead of copying the entire contents of your project directory, you can use the .dockerignore
file to exclude unnecessary files and directories, and then copy only the files that are needed for your application.
COPY package.json yarn.lock ./
RUN yarn install
COPY . .
6. Use the RUN
command to install dependencies
The RUN
command is used to execute commands in the Docker image. It's important to use it to install dependencies, rather than installing them manually on the host system. This can help ensure that your application is portable and consistent across different environments.
For example, you can use the RUN
command to install the build-essential
package, which is needed to compile C++ code.
RUN apk add --no-cache build-essential
7. Use the ENTRYPOINT
command
The ENTRYPOINT
command is used to specify the command that should be executed when the Docker container starts. This can help ensure that your application starts correctly, and it can also make it easier to manage your application in different environments.
For example, you can use the ENTRYPOINT
command to start your Node.js application.
ENTRYPOINT ["node", "dist/index.js"]
8. Use the HEALTHCHECK
command
The HEALTHCHECK
command is used to specify a command that should be executed periodically to check the health of the Docker container. This can help ensure that your application is running correctly, and it can also help you identify and troubleshoot issues more quickly.
For example, you can use the HEALTHCHECK command to check that your web server is responding to requests.
HEALTHCHECK --interval=5s --timeout=3s --retries=3 CMD curl --fail http://localhost:3000/healthz || exit 1
9. Use the USER
command
The USER
command is used to specify the user that should be used to run the Docker container. This can help improve security by reducing the privileges of the user running the container.
For example, you can use the USER
command to switch to the node
user, which has limited privileges.
USER node
10. Use the VOLUME
command
The VOLUME
command is used to specify a directory that should be used to store data outside of the Docker container. This can help improve performance and make it easier to manage your data in different environments.
For example, you can use the VOLUME
command to store your application's logs outside of the container.
VOLUME ["/app/logs"]
Conclusion
Writing a Dockerfile is an important step in creating a Docker image, and following best practices can help ensure that your images are efficient, secure, and easy to maintain. By using a minimal base image, multi-stage builds, environment variables, and other best practices, you can create Docker images that are optimized for performance and security.