Infrastructure Testing with OpenTofu and Acceptance Tests


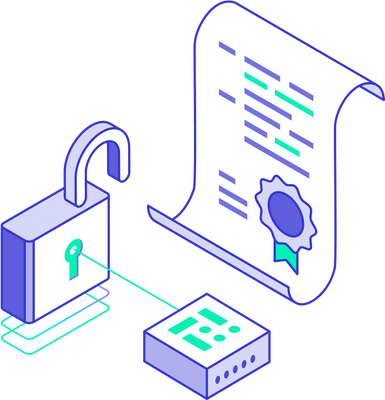
OpenTofu is an open-source tool that enables infrastructure testing by providing a framework for defining and executing tests against infrastructure as code (IaC) artifacts. It supports multiple IaC tools, including Terraform, CloudFormation, and Kubernetes. OpenTofu tests can be written in any language that supports the OpenTofu SDK, including Python, Go, and Ruby.
Acceptance tests are a type of functional test that verify that the system meets the business requirements. They are typically written from the perspective of the end-user and focus on the overall behavior of the system. Acceptance tests can be used to validate that the infrastructure is correctly configured and that the application is functioning as expected.
Let's dive into how to use OpenTofu and acceptance tests to perform infrastructure testing.
Setting up OpenTofu
To get started with OpenTofu, you'll need to install the OpenTofu CLI and SDK. The CLI is used to run tests, while the SDK is used to write tests. You can install the CLI using pip:
sudo snap install opentofu --classic
With the SDK installed, you can create a new OpenTofu project using the tofu init
command:
tofu init myproject
This will create a new directory called myproject
with a basic project structure.
Writing OpenTofu tests
OpenTofu tests are written in a declarative language that describes the expected state of the infrastructure. Tests are organized into suites, which can be run together or individually.
Here's an example of an OpenTofu test written in HCL:
# test.tftest.hcl
run "test_ec2_instance_exists" {
module {
source = "./path/to/my/ec2/module"
}
assert {
condition = resource("aws_instance.my_instance") != null
error_message = "EC2 instance does not exist"
}
}
tofu test -f test.tftest.hcl
In this example, the test defines a Terraform module located at ./path/to/my/ec2/module
and asserts that the aws_instance.my_instance
resource exists. If the resource does not exist, the test will fail with the specified error message.
Here's an example of a more complex test that asserts that an EC2 instance has the correct security group and that it is running:
# test-running.tftest.hcl
run "test_ec2_instance_configured_correctly" {
module {
source = "./path/to/my/ec2/module"
}
# Assert that the EC2 instance exists
assert {
condition = resource("aws_instance.my_instance") != null
error_message = "EC2 instance does not exist"
}
# Assert that the EC2 instance has the correct security group
assert {
condition = contains(resource("aws_instance.my_instance").attribute("security_groups"), "my-security-group")
error_message = "EC2 instance does not have the correct security group"
}
# Assert that the EC2 instance is running
assert {
condition = resource("aws_instance.my_instance").attribute("instance_state") == "running"
error_message = "EC2 instance is not running"
}
}
tofu test -f test-running.tftest.hcl
This test asserts that the aws_instance.my_instance
resource exists, has the correct security group, and is running. If any of these assertions fail, the test will fail with the specified error message.
Running OpenTofu tests
Once you have written your OpenTofu tests, you can run them using the tofu run command:
tofu run myproject
This will run all the tests in the myproject
directory. You can also run individual tests or test suites using the --test
or --suite
flags, respectively.
OpenTofu provides detailed output for each test, including the test name, duration, and result. If a test fails, OpenTofu will provide information about the failure, including the assertion that failed and the expected and actual values.
Integrating OpenTofu with CI/CD
To ensure that your infrastructure is tested consistently, it's crucial to integrate OpenTofu with your CI/CD pipeline. This integration can be achieved using the OpenTofu CLI and a CI/CD tool such as Jenkins, CircleCI, or GitHub Actions.
Here's an example of a GitHub Actions workflow that runs OpenTofu tests as part of a pull request:
name: OpenTofu Tests
on: [pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Installing OpenTofu CLI
uses: opentofu/setup-opentofu@v1
with:
tofu_version: 1.6.0
- name: Run OpenTofu tests
run: |
tofu test -f test.tftest.hcl
tofu test -f test-running.tftest.hcl
This workflow runs the OpenTofu tests whenever a pull request is opened or updated. If any of the tests fail, the workflow will fail, and the pull request will not be merged.
Using acceptance tests to validate infrastructure
While OpenTofu tests are useful for validating the state of the infrastructure, they do not necessarily validate that the infrastructure meets the business requirements. Acceptance tests can be used to validate that the infrastructure is correctly configured and that the application is functioning as expected.
Acceptance tests can be written using a tool such as Behave or Cucumber. These tools provide a way to write tests in a natural language that is easy for non-technical stakeholders to understand.
Here's an example of an acceptance test written using Behave:
Feature: EC2 instance
Scenario: EC2 instance is running
Given an EC2 instance with name "my-instance"
When I check the instance status
Then the instance should be "my-security-group"
This acceptance test defines two scenarios: one that checks that the EC2 instance is running, and another that checks that the EC2 instance has the correct security group.
To run these acceptance tests, you can use a tool such as Behave or Cucumber to execute the tests and validate the results. These tools provide a way to execute the tests and generate reports that can be shared with stakeholders.
Integrating acceptance tests with OpenTofu
While OpenTofu and acceptance tests serve different purposes, they can be integrated to provide a more comprehensive testing strategy.
One way to integrate OpenTofu and acceptance tests is to use OpenTofu to provision the infrastructure and then use acceptance tests to validate that the infrastructure is correctly configured and that the application is functioning as expected.
Here's an example of how this might work:
1. Write OpenTofu tests to validate the state of the infrastructure.
2. Use OpenTofu to provision the infrastructure.
3. Use acceptance tests to validate that the infrastructure is correctly configured and that the application is functioning as expected.
4. If any of the acceptance tests fail, update the OpenTofu tests to reflect the new requirements and repeat the process.
By integrating OpenTofu and acceptance tests in this way, you can ensure that your infrastructure is tested comprehensively and that it meets the business requirements.
Conclusion
Infrastructure testing is a critical aspect of modern software development. OpenTofu provides a powerful framework for testing infrastructure as code artifacts, while acceptance tests can be used to validate that the infrastructure meets the business requirements. By integrating OpenTofu and acceptance tests, you can ensure that your infrastructure is tested comprehensively and that it is reliable, scalable, and secure.
Acceptance Tests with OpenTofu: Acceptance tests are a crucial aspect of infrastructure testing, as they ensure that the deployed infrastructure meets the required specifications and functions as expected. OpenTofu's testing framework is designed to support acceptance tests, allowing users to define assertions that check the state of the infrastructure after the apply
or plan
operation. These assertions can be used to verify the correctness of the infrastructure, such as checking the ARN value of an AWS S3 bucket.
Complexities and Challenges: While OpenTofu's testing framework offers a robust approach to infrastructure testing, there are complexities and challenges to consider. One of the main challenges is the need to balance the scope and granularity of tests, as higher-level tests can be time-consuming and costly. Setting up and tearing down resources for integration tests can be a lengthy process, making it essential to implement unit and contract testing to fail quickly on wrong configurations.